Hola, continuemos con el tutorial CRUD con MVP (Parte 2). Hasta ahora tenemos la base del patrón MVP, ahora, para que la aplicación funcione, implementaremos los repositorios de la capa de acceso a datos.
Tutorial
Código
Repositorio
BaseRepository.cs
public abstract class BaseRepository { protected string connectionString; //... }
PetRepository.cs
public class PetRepository : BaseRepository, IPetRepository { //Constructor public PetRepository(string connectionString) { this.connectionString = connectionString; } //Methods public IEnumerable<PetModel> GetAll() { var petList = new List<PetModel>(); using (var connection = new SqlConnection(connectionString)) using (var command = new SqlCommand()) { connection.Open(); command.Connection = connection; command.CommandText = "Select *from Pet order by Pet_Id desc"; using (var reader = command.ExecuteReader()) { while (reader.Read()) { var petModel = new PetModel(); petModel.Id = (int)reader[0]; petModel.Name = reader[1].ToString(); petModel.Type = reader[2].ToString(); petModel.Colour = reader[3].ToString(); petList.Add(petModel); } } } return petList; } public IEnumerable<PetModel> GetByValue(string value) { var petList = new List<PetModel>(); int petId = int.TryParse(value, out _) ? Convert.ToInt32(value) : 0; string petName = value; using (var connection = new SqlConnection(connectionString)) using (var command = new SqlCommand()) { connection.Open(); command.Connection = connection; command.CommandText = @"Select *from Pet where Pet_Id=@id or Pet_Name like @name+'%' order by Pet_Id desc"; command.Parameters.Add("@id", SqlDbType.Int).Value = petId; command.Parameters.Add("@name", SqlDbType.NVarChar).Value = petName; using (var reader = command.ExecuteReader()) { while (reader.Read()) { var petModel = new PetModel(); petModel.Id = (int)reader[0]; petModel.Name = reader[1].ToString(); petModel.Type = reader[2].ToString(); petModel.Colour = reader[3].ToString(); petList.Add(petModel); } } } return petList; } public void Add(PetModel petModel) { throw new NotImplementedException(); } public void Delete(int id) { throw new NotImplementedException(); } public void Edit(PetModel petModel) { throw new NotImplementedException(); } }
Cadena de conexion (App.config)
<?xml version="1.0" encoding="utf-8" ?> <configuration> <configSections> </configSections> <connectionStrings> <add name="SqlConnection" connectionString="Data Source=(local);Initial Catalog=VeterinaryDb;Integrated Security=True" providerName="System.Data.SqlClient" /> </connectionStrings> <startup> <supportedRuntime version="v4.0" sku=".NETFramework,Version=v4.5" /> </startup> </configuration>
Vista
IMainView.cs (Interfaz)
public interface IMainView { event EventHandler ShowPetView; event EventHandler ShowOwnerView; event EventHandler ShowVetsView; }
MainView.cs (Diseño)
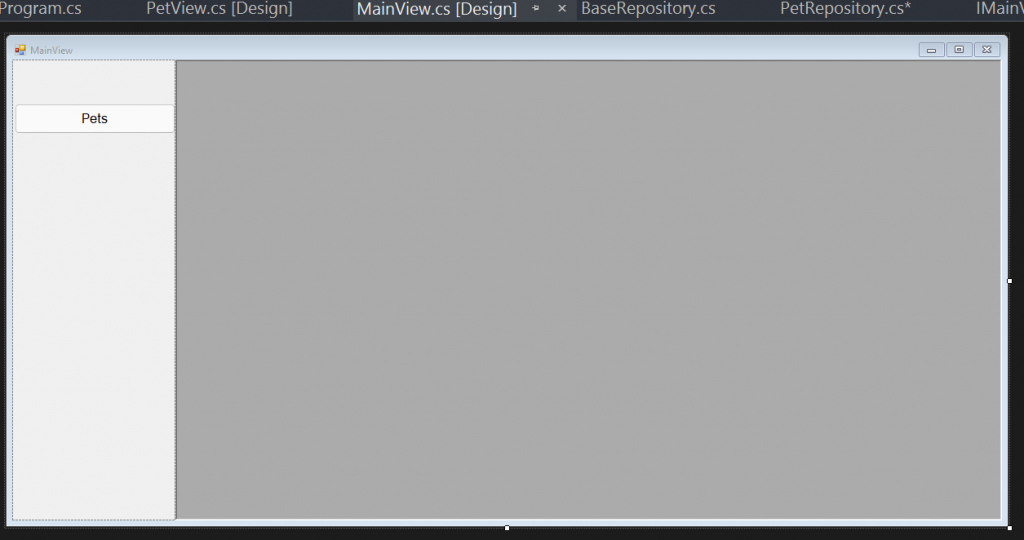
MainView.cs (Código)
public partial class MainView : Form, IMainView { public MainView() { InitializeComponent(); btnPets.Click += delegate { ShowPetView?.Invoke(this, EventArgs.Empty); }; } public event EventHandler ShowPetView; public event EventHandler ShowOwnerView; public event EventHandler ShowVetsView; }
Presentador
MainPresenter.cs
public class MainPresenter { private IMainView mainView; private readonly string sqlConnectionString; public MainPresenter(IMainView mainView, string sqlConnectionString) { this.mainView = mainView; this.sqlConnectionString = sqlConnectionString; this.mainView.ShowPetView += ShowPetsView; } private void ShowPetsView(object sender, EventArgs e) { IPetView view = PetView.GetInstace((MainView)mainView); IPetRepository repository = new PetRepository(sqlConnectionString); new PetPresenter(view, repository); } }
Clase Program (Ejecutar aplicación)
static class Program { [STAThread] static void Main() { Application.EnableVisualStyles(); Application.SetCompatibleTextRenderingDefault(false); string sqlConnectionString = ConfigurationManager.ConnectionStrings["SqlConnection"].ConnectionString; IMainView view = new MainView(); new MainPresenter(view,sqlConnectionString); Application.Run((Form)view); } }